Firebase is a great platform for developing rich mobile and web applications by leveraging the power and scale of the Google Cloud Platform. Firebase provides developers with a wide range of composable tools, backend services, and SDKs to help them develop high-quality apps with user authentication, Performance Monitoring, Real time Database, and many more. In this article I’ll be sharing a few simple and easy to implement Firebase security rules.
Firebase Authentication
Have you ever noticed that when we build a Firebase app we can perform user Authentication and works with our database entirely from the front-end side? But how is this possible if our database is exposed to the client-side? wouldn’t that mean that any hacker or third-party could be able to read or write our private user data?
Technically, The answer is NO, because the whole infrastructure is protected by a mechanism known as Firebase Security which stands in between our data and the user. These rules are hosted on Firebase servers and are applied automatically at all times. We can also write simple or complex rules that protect our app’s data to the level of security that our app specifically requires. These security rules can be applied to secure our data in Cloud Firestore, Firebase Real time Database, and everything in Cloud Storage.
Using Firebase Security Rules
In a traditional web application, we have a server and on that server we implement security rules. The logic uses either cookies or tokens to verify that a user requests to check whether the user is authorized to do so. With the help of Firebase security rules, we can achieve the same, but without the need to write or maintain and deploy server-side code for security aspects. Instead, we can create a policy to define who has access to what all data in our database. This can be done by a very easy to learn JavaScript-like syntax language called common expression language.
Whenever, the user makes a request to read/write in our database, that request is routed through this policy. When a request comes in, it will look for the first rule to allow it and, once allowed Firebase can then securely read or write to our database.

We can write rules from the Firebase console or from our IDE in the console. We can also time travel back to any previous rules we’ve used in the past or use the playground to send a mock request to your database and in production, where we can analyze exactly how your Firebase security rules are being executed.
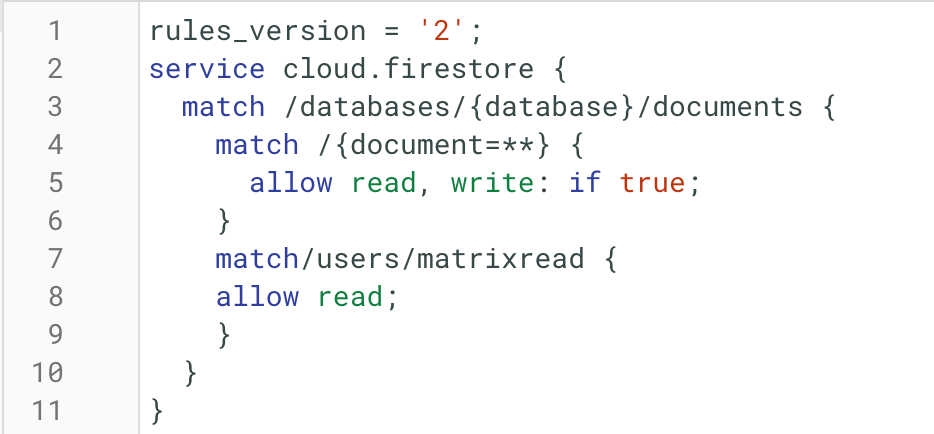
Types of Firebase Real time Database Rules we can use in our Projects,
No Security
As the name suggests, this one comes with the least security or literally no security even people who are not users of our app, will get access to our database and can do read and write operations. This one is quite useful while prototyping as it bypasses authentication. But before launching we should make sure to configure some basic security rules to prevent any misconduct.
Full Security
Here we can access the database only through the Firebase console. These are the default rules that disable read and write access to our database by users.
Authentication of the user from a particular domain
These rules give access to the user from a particular domain (@example.com). The below code shows how this rule give access to members of matrixread.
{
“rules”: {
“.read”: “auth.token.email.endsWith(‘@matrixread.com’)”,
“.write”: “auth.token.email.endsWith(‘@matrixread.com’)”
}
}
User Data Only
Consider we are building a learning management system app. Here we need to have 2 different logins one for students and the other for professors. We can use the User Data Only rule which gives each authenticated user a personal node at /post/$user_id
where $user_id
is the ID of the user obtained through Authentication. So accordingly each user would be able to see their private data. Lets under the above rule with the help of the code.
{
"rules": {
"users": {
"$uid": {
".read": "$uid === auth.uid",
".write": "$uid === auth.uid"
}
}
}
}
Conclusion
I’m really amazed by the simplicity and solid security options of Firebase but this is only the beginning, there are many options to implement personalized security rules, we can even create and apply our own security rules its just that the queries must follow the constraints set by our own security rules.